本文最后更新于:1 年前
2.2 实现如下的程序功能(4 学时)
内容:
1、使用 ListView 实现如下程序界面和功能,如图 4 所示。
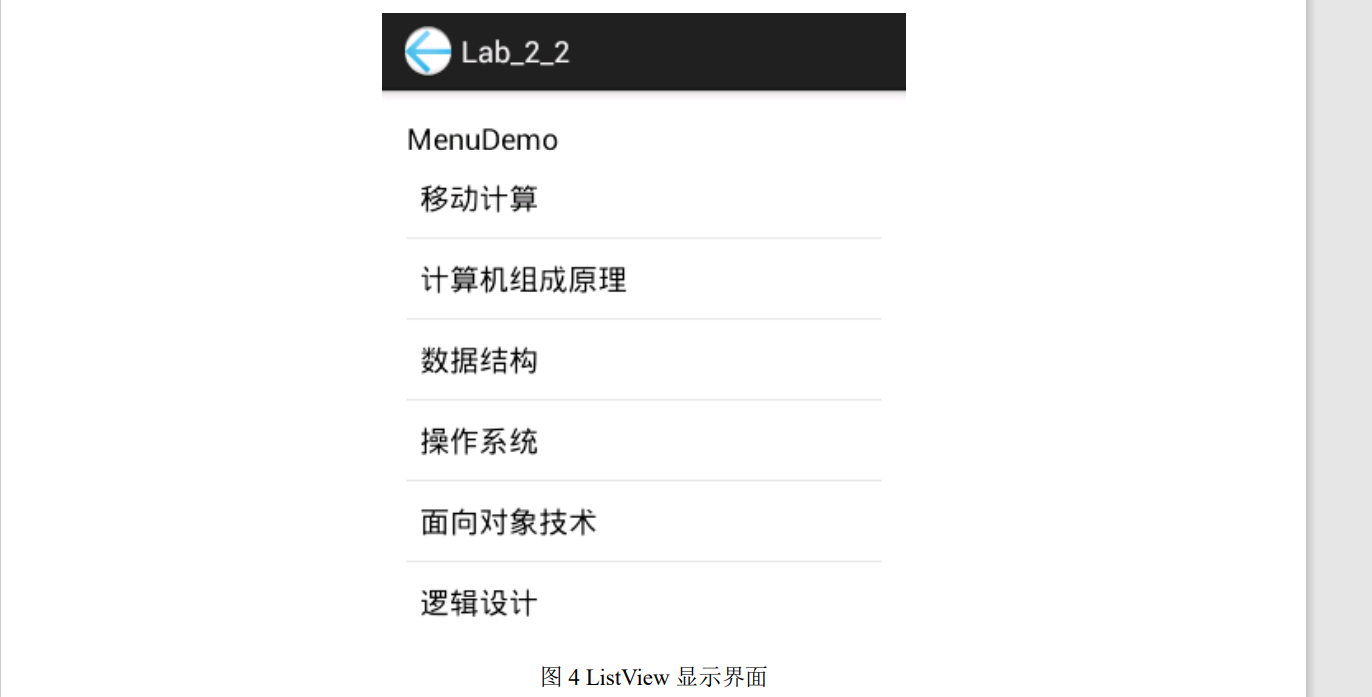
2、当选择某一 ListView 子项,TextView(即 MenuDemo 位置)中会显示所选子项内容,如图 5 所示。
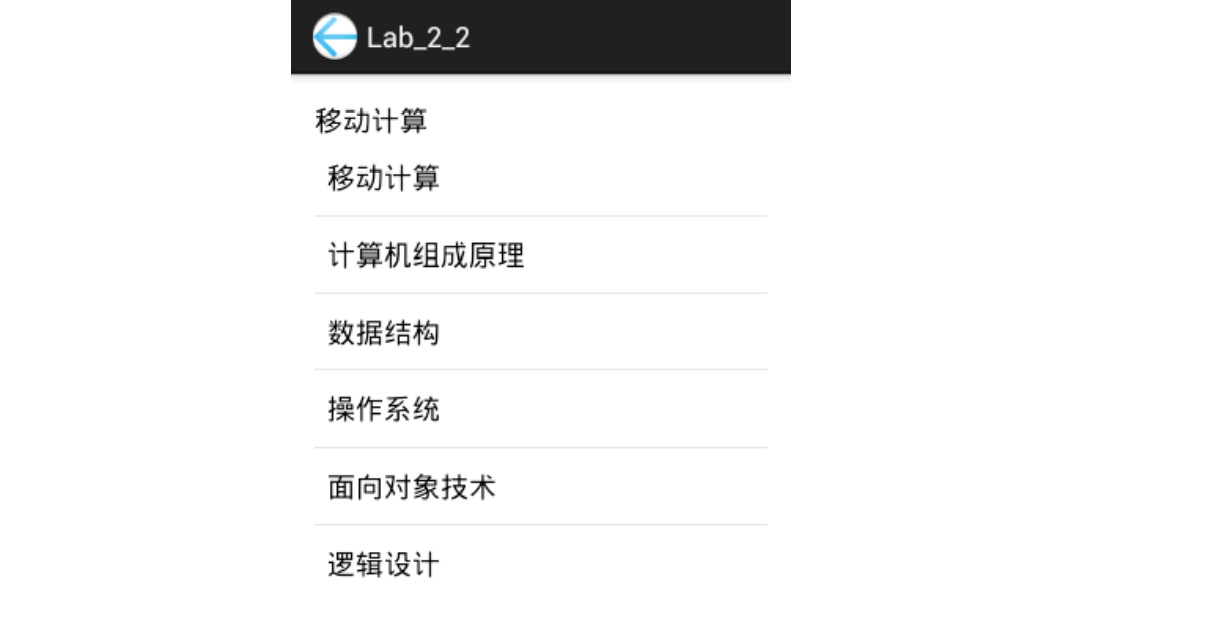
3、采用自定义布局 BaseAdapter 修改列表颜色,如图 6 所示。
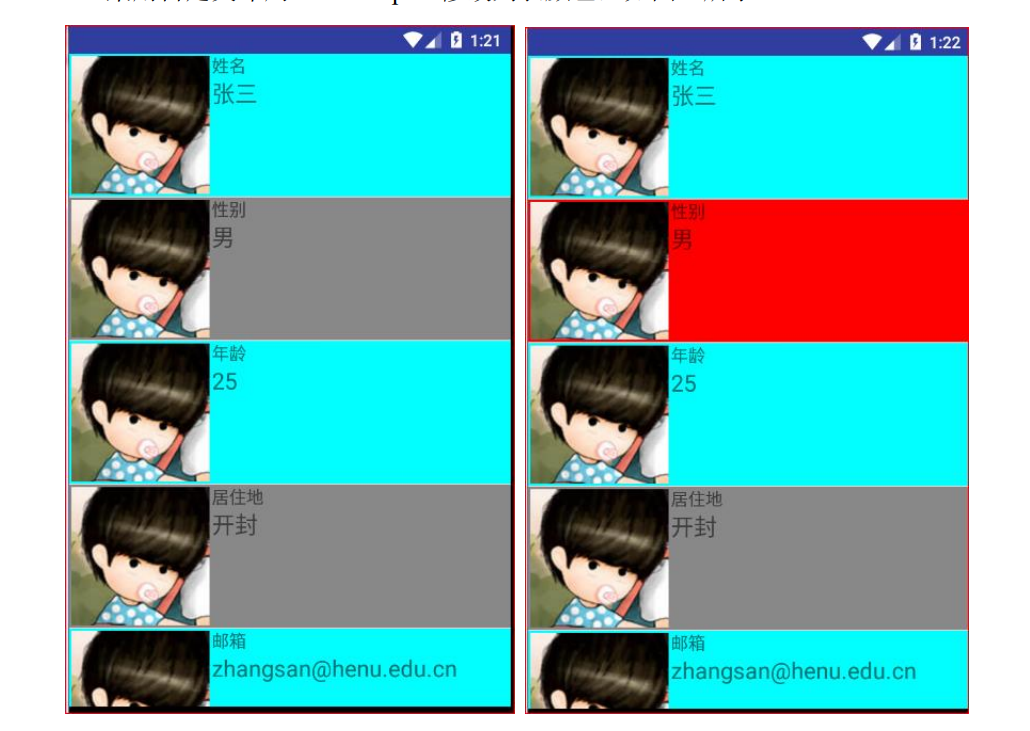
实验步骤:
1、 先建立一个空工程,在activity_main.xml文件中写入Textview和ListView。
2、 在Mainactivity.java文件中创建listview数组,编写点击listview事件,修改textView里面文字的内容。
3、 在activity_main.xml文件里编写一个按钮,在主函数中编写点击按钮跳转界面,用intent函数,跳入lab2_2_2.java;
4、 建立lab2_2_2.xml文件。编写一个listview
5、 建立item.xml文件,编写一个listview里面的内容的布局。
6、 自定义一个Person类,建立name,des,header等函数。
7、 自定义MyBaseAdapter类,继承BaseAdapter,编写里面的getview方法,在里面修改listview的颜色
代码如下:
activity_main.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:orientation="vertical" android:layout_height="match_parent" tools:context=".MainActivity" xmlns:android="http://schemas.android.com/apk/res/android">
<TextView android:id="@+id/textView" android:layout_width="match_parent" android:layout_height="40dp" android:text="MenuDemo" android:textSize="24sp" />
<ListView android:id="@+id/list1" android:layout_width="match_parent" android:layout_height="400dp" /> <Button android:id="@+id/tiaozhuan" android:layout_width="wrap_content" android:text="跳转" android:layout_height="wrap_content">
</Button> </LinearLayout>
|
item.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="horizontal">
<ImageView android:id="@+id/header" android:layout_width="wrap_content" android:layout_height="wrap_content" />
<LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical">
<TextView android:id="@+id/name" android:layout_width="match_parent" android:layout_height="wrap_content" />
<TextView android:id="@+id/des" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout> </LinearLayout>
|
lab2_2_2.xml
version1 2 3 4 5 6 7 8 9 10 11 12 13
| <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:orientation="horizontal" android:layout_height="match_parent"> <ListView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/mylistview" android:divider="@color/purple_500" android:dividerHeight="3dp" />
</LinearLayout>
|
MainActuvuty.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| package com.example.lab2_2;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.ArrayAdapter; import android.widget.Button; import android.widget.ListView; import android.widget.TextView;
public class MainActivity extends AppCompatActivity { private String []data={"移动计算","计算机组成原理","数据结构","操作系统","面向对象","逻辑设计",}; private ListView listView; private TextView textView; private Button btn1; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); this.textView=findViewById(R.id.textView); this.btn1=findViewById(R.id.tiaozhuan); this.listView=super.findViewById(R.id.list1); this.listView.setAdapter(new ArrayAdapter<String>(this, android.R.layout.simple_expandable_list_item_1,this.data)); listView.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) { textView.setText(data[i]); } }); btn1.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Intent intent=new Intent(MainActivity.this,lab2_2.class); startActivity(intent);
} });
} }
|
MyBaseAdapter.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| package com.example.lab2_2;
import android.annotation.SuppressLint; import android.app.Activity; import android.app.ListActivity; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.ListView; import android.widget.SimpleAdapter; import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map;
public class lab2_2 extends AppCompatActivity { ListView mylistview;
private List<Map<String, Object>> listsimple = new ArrayList(); private int[] headers = {R.drawable.ic_launcher_foreground, R.drawable.ic_launcher_foreground, R.drawable.ic_launcher_foreground}; private List<People> list=new ArrayList<>(); @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.lab2_2_2); mylistview = findViewById(R.id.mylistview); for (int i = 0; i < 10; i++) { People p=new People("2012020019","张志豪",headers[0]); list.add(p); } MyBaseAdapter simpleAdapter=new MyBaseAdapter(this,list); mylistview.setAdapter(simpleAdapter); mylistview.setOnItemClickListener(new AdapterView.OnItemClickListener() { @SuppressLint("ResourceAsColor") @Override
public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) { simpleAdapter.setIndex(i); simpleAdapter.notifyDataSetChanged(); } }); }
}
|
people.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| package com.example.lab2_2; public class People { private String name; private String des; private int header;
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public String getDes() { return des; }
public void setDes(String des) { this.des = des; }
public int getHeader() { return header; }
public void setHeader(int header) { this.header = header; }
public People() {
}
public People(String des, String name, int header) { this.name = name; this.des = des; this.header = header; } }
|
lab2_2.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| package com.example.lab2_2;
import android.app.Activity; import android.app.ListActivity; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.ListView; import android.widget.SimpleAdapter; import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map;
public class lab2_2 extends AppCompatActivity { ListView mylistview;
private List<Map<String, Object>> listsimple = new ArrayList(); private int[] headers = {R.drawable.ic_launcher_foreground, R.drawable.ic_launcher_foreground, R.drawable.ic_launcher_foreground}; private List<People> list=new ArrayList<>(); @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.lab2_2_2); mylistview = findViewById(R.id.mylistview); for (int i = 0; i < 10; i++) { People p=new People("2012020019","张志豪",headers[0]); list.add(p); } MyBaseAdapter simpleAdapter=new MyBaseAdapter(this,list); mylistview.setAdapter(simpleAdapter); }
}
|
问题讨论:
1、问题:MainActivity不知道怎么获取listview里position的值,当时用的是listview.setonclicklistener,
解决办法:后来在网上查询可知,用listview.setOnItemClickListener,获取里面的position,然后用textView.setText(data[i])来替换Textview里面的值。
2、问题:在第一个界面写一个按钮,跳转到第二个界面,写lab2_2_2,在Mainactivity用intent跳转,跳转写完之后,启动界面没有反应,
解决方法:在AndroidManifest.xml文件中注册一个活动
在上面加入
活动,就能成功跳转。
3、问题:图文列表建立后不知道怎么给listview上不同的颜色。
解决办法:我是用书上给的方法,后来借鉴老师上课讲的代码,又重新写了一下,重写BaseAdapter,建立people类,建立item.xml文件,在getview()里面用i的奇偶来给listview上不同的颜色。