本文最后更新于:1 年前
实验内容
- 使用 Intent 启动 Activity 的方法
- 获取 Activity 返回值的方法
- 发送和接收广播消息的方法
实验目的和要求
- 了解使用 Intent 进行组件通信的原理
- 掌握使用 Intent 启动 Activity 的两种方式
- 掌握获取 Activity 返回值的方法
- 了解 Intent 过滤器的原理与匹配机制
- 掌握发送和接收广播消息的方法
实验步骤
3.1 实现如下的程序功能
1、程序主界面如图 1 所示,显示一个人名列表。要求人列表中必须包含你自己的名字。
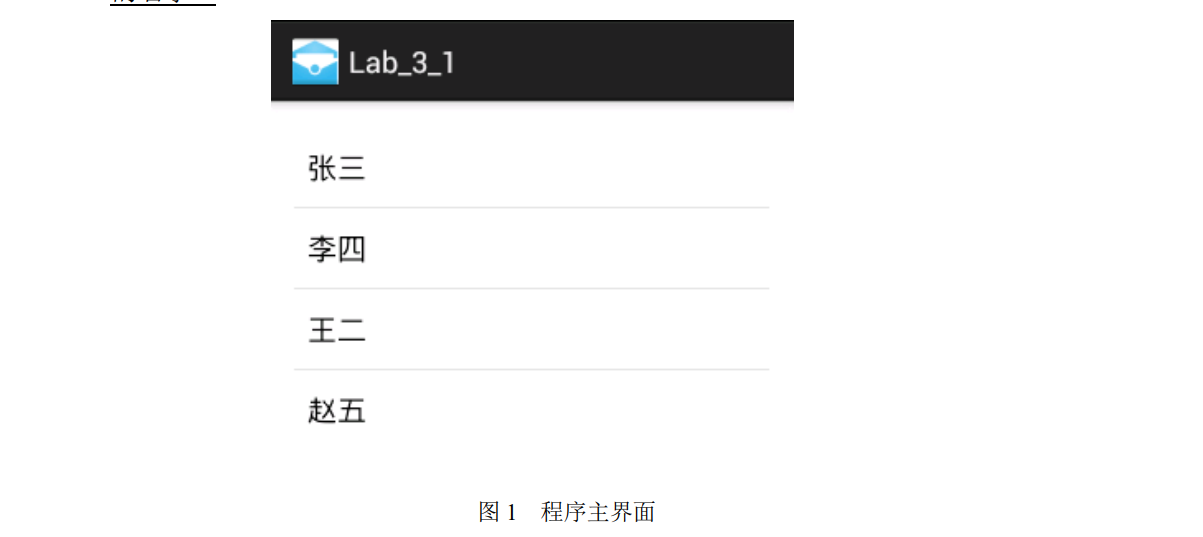
2、当用户点击列表中的某一项目时,启动一个确认删除相应记录的子 Activity,如图 2、图 3 所示。
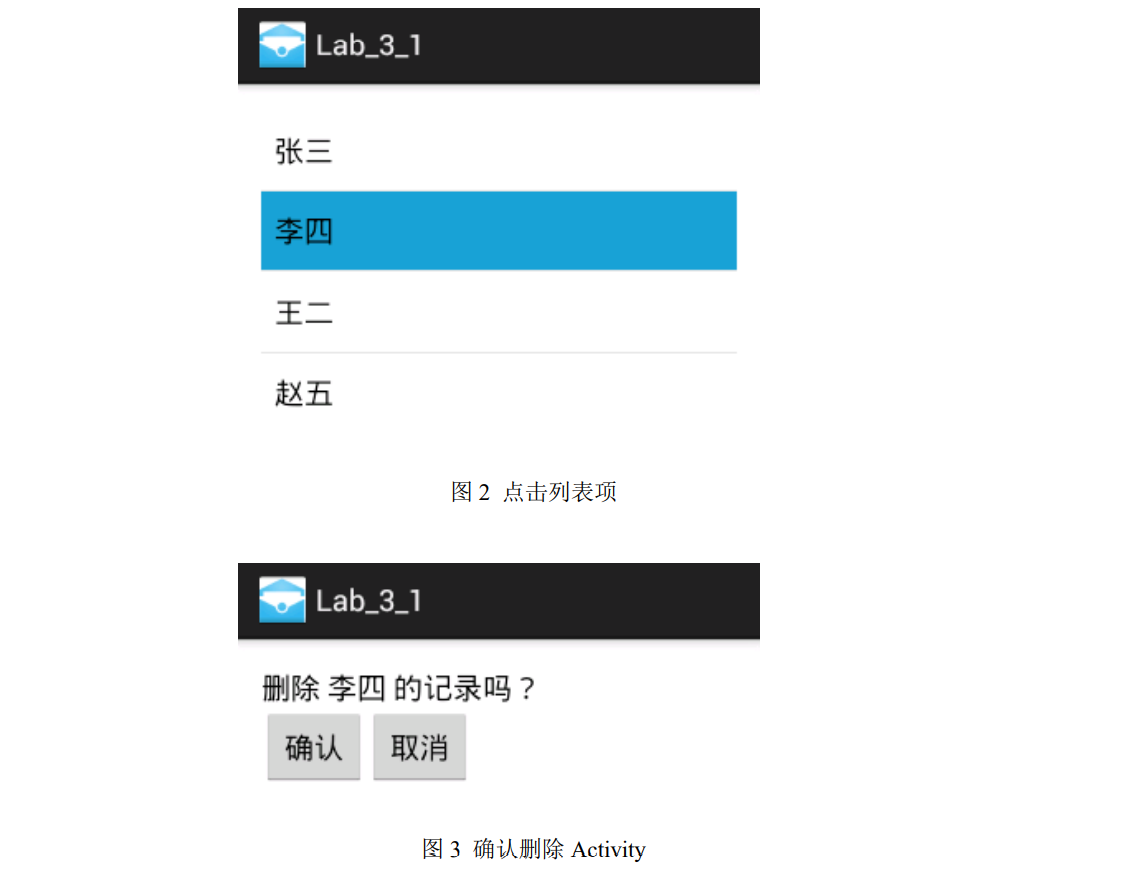
3、子 Activity 提供了提示信息,以及“确认”和“取消”两个按钮。如果点击
“确认”按钮,则在返回父 Activity 界面后删除对应列表条目;如果用户点击“取
消”按钮,则返回父 Activity 后没有任何动作。
实验步骤:
1、创建工程。
2、在activity_main.xml文件中编写listview。
3、在Mainactivity.java中创建String类型的data数组。
4、创建Arraylist list变量,用来存放listview中的内容。
5、将data数组放入list中。
6、定义调用Arrayadapter函数,将list中的内容传递到界面。
7、编写listview点击事件,点击后实现跳转,并把跳转后的值传递到另一个界面。
8、创建并编写delete.xml,在其中放入一个textview和两个按钮。
9、创建并编写delete.java文件,在其中接收MainActivity.java传来的值,并改变textview中显示的内容。
10、编写确定和取消按钮的跳转事件,并传递值。
11、在AndroidManifest.xml文件中注册delete.java文件。
12、在MainActivity.java文件中编写函数,如果点击确定,则移除list中的值。
实验代码
activity_xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:orientation="vertical" android:layout_height="match_parent" tools:context=".MainActivity"> <ListView android:id="@+id/listview" android:layout_width="match_parent" android:layout_height="600dp">
</ListView>
</LinearLayout>
|
delete.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:orientation="vertical" android:layout_height="match_parent">
<TextView android:id="@+id/textview" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="删除 的聊天记录" android:textSize="30sp">
</TextView> <LinearLayout android:layout_width="match_parent" android:orientation="horizontal" android:layout_height="wrap_content"> <Button android:id="@+id/btn1" android:text="确认" android:layout_marginLeft="27dp" android:layout_width="wrap_content" android:layout_height="wrap_content">
</Button>
<Button android:id="@+id/btn2" android:layout_marginLeft="30dp" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="取消">
</Button>
</LinearLayout>
</LinearLayout>
|
MainActivity.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60
| package com.example.lab3;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.ArrayAdapter; import android.widget.ListView; import android.widget.SimpleAdapter;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity { static String [] data={"张三","李四","王二","赵五",,"zzh"}; ListView listView=null; static int position=-1,flag=-1; ArrayList<String> list; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); list = new ArrayList<String>(); setContentView(R.layout.activity_main); listView=findViewById(R.id.listview); for(int i=0;i<data.length;i++) { if(data[i]!=" ") list.add(data[i]); } ArrayAdapter listadapter=new ArrayAdapter<String>(this, android.R.layout.simple_expandable_list_item_1,this.list); listView.setAdapter(listadapter);
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) { position=i; Intent intent=new Intent(MainActivity.this,delete.class); intent.putExtra("list",list.get(i)); startActivity(intent); } }); Intent intent1=getIntent(); int cnt=0; flag=intent1.getIntExtra("flag",0); if(flag==1){ for(int i=0;i<data.length;i++) { if(data[i]==" ")continue; if(cnt==position) { list.remove(position); data[i] = " "; break; } cnt++; } listadapter.notifyDataSetChanged(); }
} }
|
delect.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48
| package com.example.lab3;
import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import java.util.ArrayList;
public class delete extends AppCompatActivity { ArrayList<String> list; String name=null; TextView textView=null; Button btn1,btn2; public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState); setContentView(R.layout.delete); textView=findViewById(R.id.textview); Intent intent=getIntent(); name=intent.getStringExtra("list"); textView.setText("删除 "+name+" 的记录吗?"); btn1=findViewById(R.id.btn1); btn2=findViewById(R.id.btn2); btn2.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Intent intent1=new Intent(delete.this,MainActivity.class); startActivity(intent1); } }); btn1.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Intent intent2=new Intent(delete.this,MainActivity.class); intent2.putExtra("flag",1); startActivity(intent2);
} });
} }
|
问题讨论:
问题:
开始写时,就用了一个data数组,来传递值,但是不知到怎么删除数组中的元素。
解决办法:
在网上查了一下,创建ArrayList list ,将data中的值赋值给它,用.remove()方法删除。所以在MainActivity.java函数中创建ArrayList list,将传到delete.java中的值也用list[i]代替。
问题:
每次点击确定后,列表能删除,但是就删除了一个,当想删除下一个时,上一个删除的又变回来了。
解决办法:
从delete.java中传递一个int 型的flag标志位,当点击确定时,移除list的同时,将data[position]中值=” ”;然后再改变从data移入list的方法,加一个判断条件,如果data[i]!=” ”;则放入其中。
构思之后,写上代码,发现没有什么效果,分析了一下,在data[]数组前加一个static变量。这样就成功了。